Division by zero has no meaning and therefore is undefined. However, if you work with data, division by zero might occur. In this article, we discuss how to avoid division by zero in SAS.
Although division by zero doesn’t make sense in the real world, SAS doesn’t complain about it. SAS just executes your code and, if a division by 0 is detected, it returns a missing value. However, SAS also writes a “NOTE: Division by zero detected at line XX column XX” to the log.

Normally, you won’t check all the notes in the log after executing your SAS code. However, if many divisions by 0 are detected, SAS writes a WARNING to the log file.

While many SAS users only check the SAS log if an ERROR occurs, good SAS Programmers do take care of WARNINGS as well and thus try to avoid division by zero.
So, how do you avoid division by zero in SAS? You can avoid division by zero in two ways. First, you can use an IF-ELSE Statement. Second, you can use the DIVIDE function. In this article, we discuss two ways how to do this.
We use the following sample data in the examples.
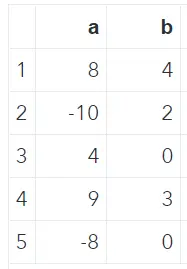
Avoid Division by Zero with IF-ELSE THEN Statement
The first option to avoid a division by 0 is by making use of an IF-ELSE THEN statement. First, we check if the denominator is zero and, if so, return a missing value. Otherwise, we return the result of the division.
data work.option_1; set work.my_ds; if b = 0 then a_over_b = .; else a_over_b =a/b; run;
SAS doesn’t create any NOTE in the log and carries out the division if possible.
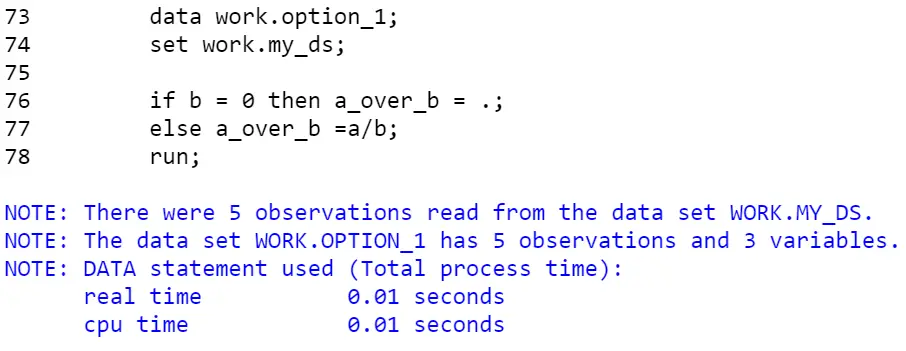
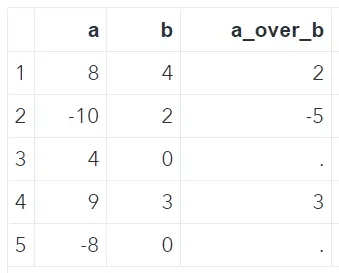
Avoid Division by Zero with DIVIDE Function
The second option to avoid division by 0 is by making use of the DIVIDE function. This function has two arguments, namely a (the nominator) and b the (denominator), and returns the result of a/b. If b is zero, the DIVIDE function doesn’t write NOTE to the log. Instead, it returns I (plus-infinity) or M (minus-infinity), depending on the value of a. See the example below.
data work.option_2; set work.my_ds; a_over_b = divide(a,b); run;
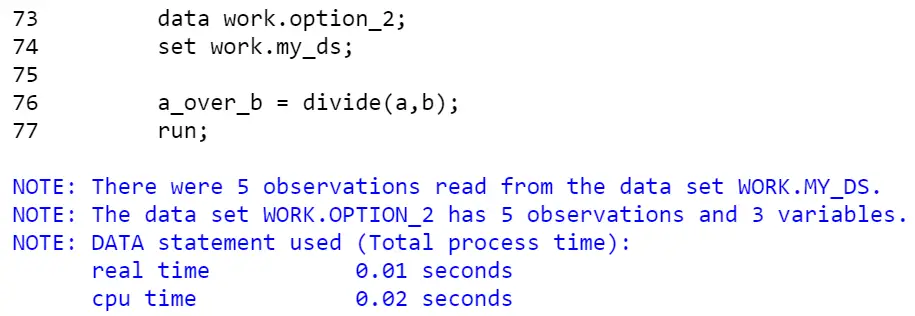
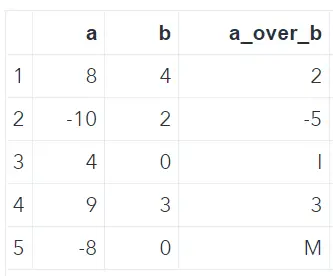
The image above demonstrates that the DIVIDE function returns I (plus-infinity) if you try to divide 4 by 0, and M (minus-infinity) if you divide -8 by 0.
Although I and M sound like “numbers”, they are instead missing values. The code below proves this with the MISSING function (where 1 is True and 0 is False).
data work.check_missing; set work.option_2; is_missing = missing(a_over_b); run;
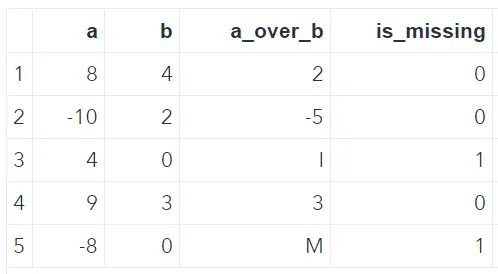