A SAS time variable (i.e., a timestamp) is a numeric variable. Its value represents the number of seconds between January 1, 1960, and a specific time. Because of this way, it makes calculations easier. But, how do you actually calculate the difference between two timestamps in SAS?
In SAS, you use the INTCK function to calculate the difference between two timestamps. You provide the start time, the end time, and the desired interval, and the INTCK function returns the difference in seconds, minutes, or hours. The INTCK function works both with time variables and datetime variables.
Besides the INTCK function, we show also another method to calculate the differences in time. This method is less elegant, but makes it possible to calculate the difference in fractions of time intervals.
If you want to know how to use the INTCK function with date variables, I recommend this detailed article.
Calculate the Difference between Timestamps – The Easy Way
The easiest way to calculate the difference between two timestamps is with the INTCK function.
INTCK("interval", start_time, end_time)
The INTCK function has 3 mandatory arguments, namely:
- interval: With the interval argument, you define the time interval you want to use to calculate the difference. You can use (between quotation marks): second, minute, or hour.
- start_time: The start time
- end_time: The end time
With these 3 obligatory arguments, the INTCK returns the difference between the two times in the specified time interval.
Examples
In the examples below, we use the INTCK function to calculate the difference in seconds, minutes, and hours between the following timestamps.
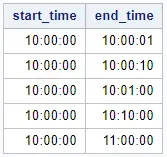
data work.my_time_ds_1; input start_time :time10. end_time :time10.; format start_time end_time time10.0; datalines; 10:00:00 10:00:01 10:00:00 10:00:10 10:00:00 10:01:00 10:00:00 10:10:00 10:00:00 11:00:00 ; run;
Difference in Seconds
You use the “second” as interval argument to calculate the difference in seconds.
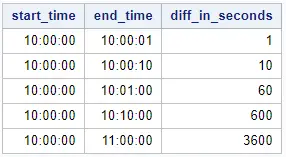
data work.difference_seconds; set work.my_time_ds_1; diff_in_seconds = intck("second", start_time, end_time); run;
Difference in Minutes
You use the “minute” as interval argument to calculate the difference in seconds.
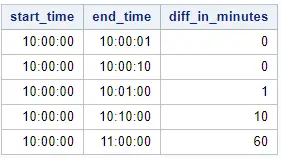
data work.difference_minutes; set work.my_time_ds_1; diff_in_minutes = intck("minute", start_time, end_time); run;
Difference in Hours
You use the “hour” as interval argument to calculate the difference in seconds.
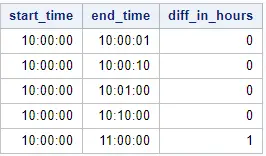
data work.difference_hours; set work.my_time_ds_1; diff_in_hours = intck("hour", start_time, end_time); run;
Remarks
- The arguments start_time and end_time must be of the same type. In other words, either both time variables or both datetime variables. In this article, I explain how to extract the time portion of a datetime variable.
- If you use the INTCK function in a macro function, the interval argument shouldn’t be between quotation marks.
Calculate the Difference between Timestamps – Discrete vs. Continuous
Suppose you have the timestamps 10:00:30AM and 10:01:00AM. Depending on your needs, the difference between both timestamps is 1 minute or 0 minutes. For this type of situation, the INTCK has a fourth (optional) argument, namely method.
The method argument can be discrete (default value) or continuous. The discrete method counts time intervals based on the boundaries of the start_time and end_time. In contrast, the continuous method shifts the start_time to count intervals.
INTCK("interval", start_time, end_time, "method")
Examples
The examples below show how to use the method argument and how the result changes depending on its value.
Difference in Minutes – Discrete vs. Continuous
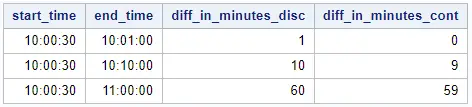
data work.my_time_ds_2; input start_time :time10. end_time :time10.; format start_time end_time time10.0; datalines; 10:00:30 10:01:00 10:00:30 10:10:00 10:00:30 11:00:00 ; run; data work.difference_minutes; set work.my_time_ds_2; diff_in_minutes_disc = intck("minute", start_time, end_time, "d"); diff_in_minutes_cont = intck("minute", start_time, end_time, "c"); run;
Difference in Hours – Discrete vs. Continuous
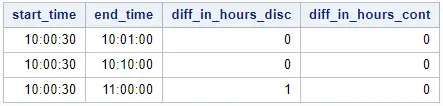
data work.difference_hours; set work.my_time_ds_2; diff_in_hours_disc = intck("hour", start_time, end_time, "d"); diff_in_hours_cont = intck("hour", start_time, end_time, "c"); run;
Calculate the Difference between Timestamps – Fractions of Time Intervals
So far, we have used the INTCK function to calculate the difference between two SAS timestamps. A drawback of the INTCK function is that it always returns an integer. However, for some reason, it might be necessary to calculate the difference with decimals. For example, 1.5 minutes.
Because time variables and datetime variables are stored as a number, it is easy to calculate the difference with fractions of intervals. You only need to subtract the start_time from the end_time and divide the result by 60 (for minutes) or 3600 (for hours).
Difference in Minutes – Fractions
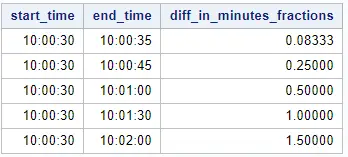
data work.my_time_ds_3; input start_time :time10. end_time :time10.; format start_time end_time time10.0; datalines; 10:00:30 10:00:35 10:00:30 10:00:45 10:00:30 10:01:00 10:00:30 10:01:30 10:00:30 10:02:00 ; run; data work.difference_minutes; set work.my_time_ds_3; diff_in_minutes_fractions = (end_time - start_time) / 60; run;
Difference in Hours – Fractions
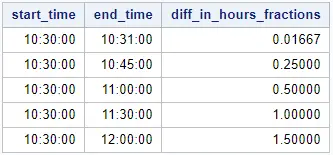
data work.my_time_ds_4; input start_time :time10. end_time :time10.; format start_time end_time time10.0; datalines; 10:30:00 10:31:00 10:30:00 10:45:00 10:30:00 11:00:00 10:30:00 11:30:00 10:30:00 12:00:00 ; run; data work.difference_hours; set work.my_time_ds_4; diff_in_hours_fractions = (end_time - start_time) / 3600; run;