Although many SAS Programmers don’t delete unneeded macro variables, you should do it. It keeps your dictionary tables clean and releases memory.
But, how do you remove macro variables in SAS? You delete SAS macro variables with the %SYMDEL statement or the CALL SYMDEL routine. Both options remove the specified macro variable(s) from the global symbol table.
In this article, we discuss how to use the %SYMDEL statement and the CALL SYMDEL routine. We show how to delete one, many, or multiple SAS macro variables.
Contents
Delete One Macro Variable
Suppose you have one macro variable, for example, my_macro_var, and you want to delete it. You can use both the %SYMDEL statement and the CALL SYMDEL routine to do so.
With the %SYMDEL Statement
Firstly, we define the macro variable my_macro_var as My Macro Variable and print it to the log. Then, we delete the macro variable with the %SYMDEL statement. Finally, we try to print the macro variable to the log again. However, because we have removed the macro variable, SAS returns a warning.
%let my_macro_var = My Macro Variable; %put &=my_macro_var.; %symdel my_macro_var; %put &=my_macro_var.;
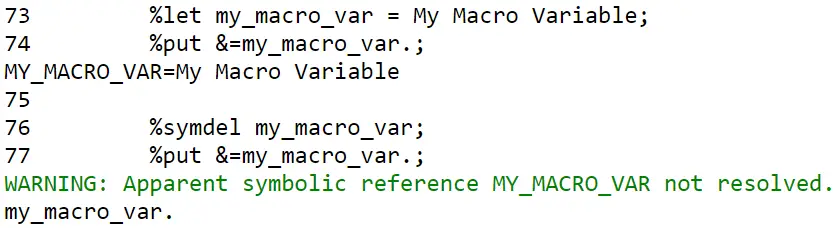
Note that, to remove the macro variable, it isn’t necessary to use the ampersand before the name of the macro variable.
With the CALL SYMDEL routine
Again, we define the macro variable my_macro_var as My Macro Variable and print it to de log. Then, we use the CALL SYMDEL routine in a data_null_ step to delete this macro variable. We confirm that the macro variable has been removed correctly by trying to print it to the log.
%let my_macro_var = My Macro Variable; %put &=my_macro_var.; data _null_; call symdel("my_macro_var"); run; %put &=my_macro_var.;
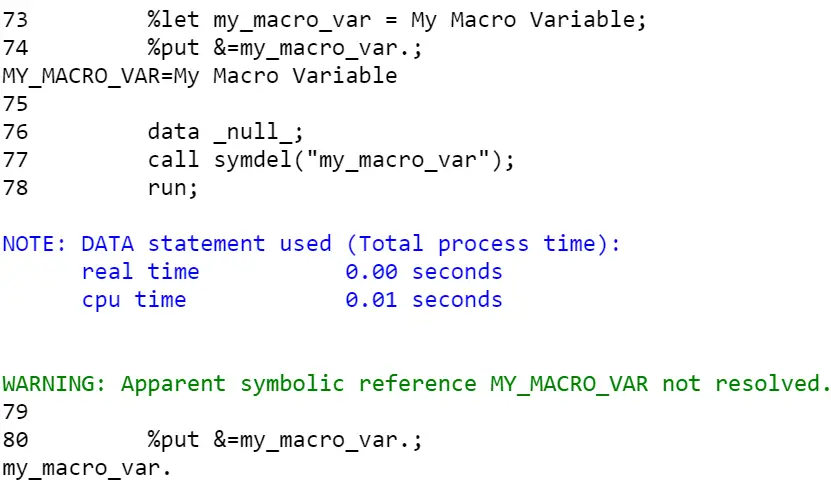
Note that, to remove a macro variable in SAS with the CALL SYMDEL routine, you need to write the name of the macro variable between quotation marks, but without the ampersand.
Delete Multiple Macro Variables
You can use both the %SYMDEL statement and the CALL SYMDEL routine to remove multiple macro variables in SAS. However, we prefer the %SYMDEL statement because this method requires less SAS code.
We create the macro variables code_one, code_two, and code_three. To remove these macro variables with the %SYMDEL statement, you need only one statement. However, if you use the CALL SYMDEL routine you need a separate routine for each variable you want to remove. See the examples below.
With the %SYMDEL Statement
%let code_one = A; %let code_two = B; %let code_three = C; %put &=code_one.; %put &=code_two.; %put &=code_three.; %symdel code_one code_two code_three; %put &=code_one.; %put &=code_two.; %put &=code_three.;
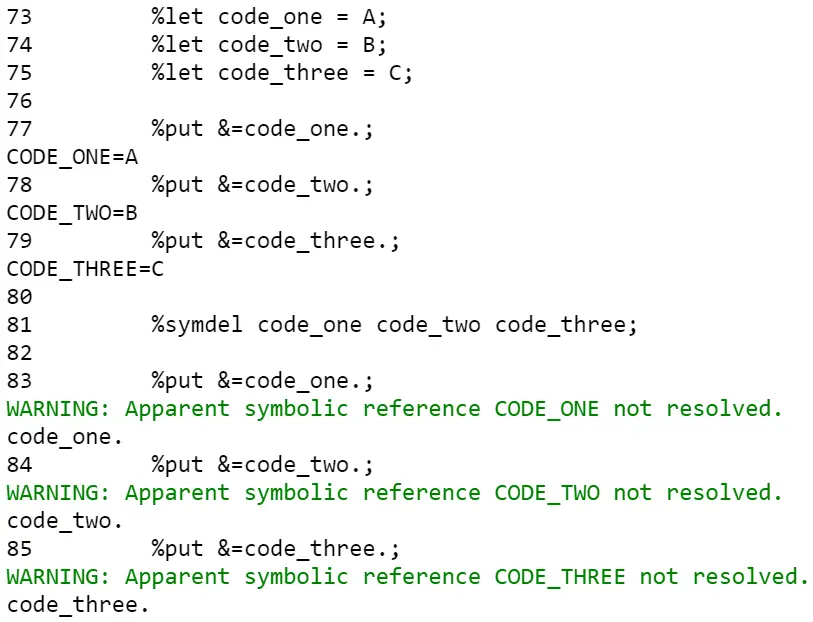
With the CALL SYMDEL routine
%let code_one = A; %let code_two = B; %let code_three = C; %put &=code_one.; %put &=code_two.; %put &=code_three.; data _null_; call symdel("code_one"); call symdel("code_two"); call symdel("code_three"); run; %put &=code_one.; %put &=code_two.; %put &=code_three.;
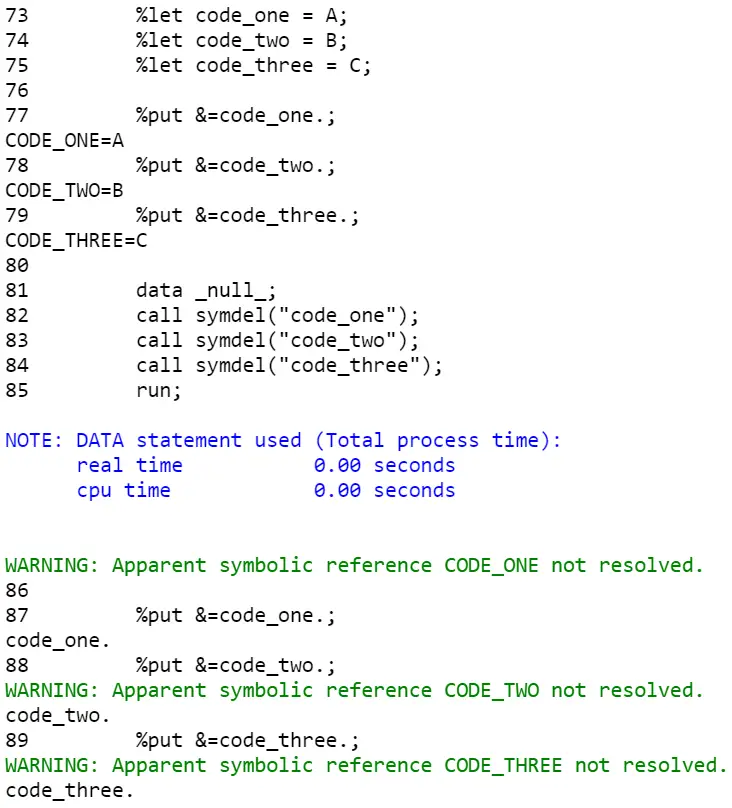
Delete Multiple Macro Variables with Similar Names
In the example above, we demonstrated how to remove the macro variables code_one, code_two, and code_three.
Although the %SYMDEL statement is the preferred option to remove multiple variables (for efficiency reasons), you would still have a hard time to delete the macro variables code_one until code_hundred because you have to write the complete variable names of 100 macro variables after the %SYMDEL statement by hand.
Here we present a macro function to delete macro variables whose names start with the same pattern.
%macro deleteSamePattern(pattern); %local macro_vars_to_delete; proc sql noprint; select name into :macro_vars_to_delete separated by " " from dictionary.macros where scope="GLOBAL" and name contains upcase("&pattern."); quit; %put variables to be deleted:; %put macro_vars_to_delete.; %symdel ¯o_vars_to_delete.; %mend deleteSamePattern; %deleteSamePattern(pattern=code_);
The macro function above consists of the following steps:
- Define a local macro variable macro_vars_to_delete.
- Use the SQL procedure to populate the local macro variable.
- The dictionary table macros in the FROM statement contains one row per macro variable that exists in the current SAS session.
- The WHERE statement filters only the macro variables that start with the desired pattern.
- The SELECT INTO clause populates the macro variable macro_vars_to_delete.
- The PUT statements show the macro variables that will be deleted.
- Use the %SYMDEL statement to delete the macro variables.
Delete All Macro Variables
Finally, suppose you want to delete all macro variables in SAS. You might think that this would work.
%SYMDELL _ALL_;
Unfortunately, this doesn’t work. So, you need a macro function to delete all your macro variables.
%macro deleteAllMacroVars(); %local macro_vars_to_delete; proc sql noprint; select name into :macro_vars_to_delete separated by " " from dictionary.macros where scope="GLOBAL" and not substr(name,1,3) = "SYS" and not name = "GRAPHTERM"; quit; %symdel ¯o_vars_to_delete.; %mend deleteAllMacroVars; %deleteAllMacroVars();
This macro function has a similar structure to the one presented in the previous section.
- Define a local macro variable macro_vars_to_delete.
- Use the SQL procedure to populate the local macro variable.
- The dictionary table macros in the FROM statement contains one row per macro variable that exists in the current SAS session.
- The WHERE statement filter all global macro variables except those that can’t be deleted.
- The SELECT INTO clause populates the macro variable macro_vars_to_delete.
- The PUT statements show the macro variables that will be deleted.
- Use the %SYMDEL statement to delete the macro variables.
Conclusions
Regarding deleting macro variables in SAS, we draw the following conclusions:
- You can use the %SYMDEL statement and the CALL SYMDEL routine to remove macro variables.
- With the %SYMDEL statement, you can delete multiple macro variables at once. With the CALL SYMDEL routine, you need a separate call for each variable.
- You can use the %SYMDEL statement as a separate statement in a SAS program or a macro function.
- You can use the CALL SYMDEL routine in a Data Step (or data _null_).
- A macro function is necessary to delete all macro variables (that start with the same pattern).
- %SYMDEL _ALL_ doesn’t work to remove all macro variables.
2 thoughts on “How to Delete User-Defined Macro Variables in SAS”
Comments are closed.