The length of your variables determines to a large extent the size of your dataset. If you specify the length correctly, you might reduce the size of your dataset by 50%. But, how do you find the length of a variable in SAS?
In SAS, the LENGTH function returns the length of a non-blank character string excluding leading and trailing blanks. The LENGTH function has one, obligatory argument, namely a character string. This string can be a variable, a constant, or an expression.
Contents
How to Find the Length of a Character Variable
As mentioned in the introduction, you use the LENGTH function to find the number of characters in a SAS variable. Here we demonstrate how to calculate the length of a character variable, a constant, and an expression.
Character Variable
We have created a SAS dataset (my_dataset) with one variable (characters) of which we will determine the length.
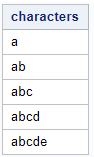
To determine the length of each value in the characters column, we use the characters column as the argument of the LENGTH function.
data work.my_dataset_length; set work.my_dataset; length_characters = length(characters); run;
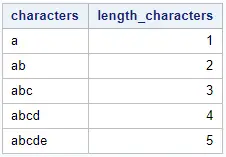
As the image above shows, the LENGTH function returns the number of characters in a SAS variable.
Character Constant
We use a Data Step to demonstrate that the LENGTH function can also return the number of characters in a constant.
data _null_; length_string_1 = length("abcdefg"); put "The number of characters in 'abcdefg' is: " length_string_1; length_string_2 = length("123%&$"); put "The number of characters in '123%&$' is: " length_string_2; run;

Character Expression
We use a Data Step and the CAT function to show that the LENGTH function can also determine the number of characters returned by an expression.
data _null_; length_string_1 = length(cat("abc", "xyz")); put "The number of characters in this expression is: " length_string_1; run;

Do you know? Count the Number of Specific Characters in a String
How to Find the Length of a Numeric Variable
Although the LENGTH function only accepts character strings as arguments, you still can use this function to determine the number of digits in a number (with some modifications).
Steps to find the length of a numeric variable in SAS (i.e., the number of digits):
- Convert the numeric value into a character value with the PUT function.
- Remove unwanted characters such as periods, dollar signs, etc. (This step might not be necessary.)
- Calculate the length with the LENGTH function.
In this example, we apply the steps mentioned above to find the number of digits in the price column.
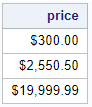
data work.price_length; set work.price; digits = length(compress(put(price, dollar12.2), , "kd")); run;
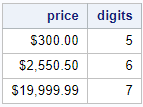
In the SAS code above, we used the COMPRESS function to keep only the digits and remove all other unwanted characters. I highly recommend reading this article which explains in more detail the COMPRESS function.
What is the Default Length of a Variable
When you create a new dataset, SAS reserves by default a length of 8 bytes to store information for each variable.
For numeric variables, 8 bytes is enough to save integers up to 16 digits.
Length in Bytes | Largest Integer Representation |
---|---|
3 | 8,192 |
4 | 2,097,152 |
5 | 536,870,912 |
6 | 137,438,953,472 |
7 | 35,184,372,088,832 |
8 | 9,007,199,254,740,990 |
As you can see, the default length in bytes that SAS reserves for numeric variables allow you to save very big integers. So, if you work with integers and you are sure that your integers won’t exceed a certain maximum, it might be worth it to change the length in bytes. (For optimization purposes.)
However, for character variables, 8 bytes correspond to just 8 characters. In other words, the length in bytes is equal to the number of characters. So, for character variables, it is normally necessary to increase the length in bytes to store all information.
How to Change the Length of a Variable
In a SAS Data Step, you can change the length in bytes of a variable with the LENGTH statement.
(Notice the difference between the LENGTH function and the LENGTH statement).
To change the length of character variables, the LENGTH statement consists of 3 steps:
- The LENGTH keyword.
- The name(s) of the variable(s) of which you want to change.
- A dollar sign followed by the desired length.
So, if you want to change the length of the column name to 25 characters, you need this LENGTH statement.
LENGTH name $25;
So, for example:
data work.ds_new; length name $25; set work.ds; run;
To change the length of multiple character columns at once, for example, name and surname, you can use this statement.
LENGTH name surname $25;
data work.ds_new; length name surname $25; set work.ds; run;
You can also use PROC SQL to change the length of a variable. However, you need to define the length of each variable one-by-one. For example:
proc sql; create table work.ds_new as select name length=25, surname length=25 from work.ds; quit;
(With PROC SQL it isn’t necessary to provide the dollar sign for character variables.)
To change the length of numeric variables, the LENGTH statement consists of 3 steps:
- The LENGTH keyword.
- The name(s) of the variable(s) of which you want to change.
- The desired length. The maximum length is 8.
What is the Maximum Length of a Variable
Finally, a frequently asked question is about the maximum length. For numeric variables the maximum length is 8. Character variables have a maximum length of 32767 in SAS.
3 thoughts on “How to Find the Length of a Variable in SAS”
Comments are closed.