You can use the INTNX function in SAS to increment a date, time, or datetime value by a given time interval.
The INTNX function has the following syntax:
INTNX(interval, start-from, increment, alignment)
where:
- interval: A date, time, or datetime interval.
- start-from: The starting date, time, or datetime.
- increment: The number of intervals to shift the start-from value.
- alignment: The position of the SAS date within an interval.
The interval argument, for example, DAY or MONTH, must be written between (double) quotes. See the official SAS documentation for a complete list of date/time intervals.
The increment argument can be a negative, positive, or zero integer. Hence, you can use the INTNX function both for adding and subtracting intervals from/to the start-from value.
The alignment argument specifies the position of a SAS date within the given interval. For example, in the case of a monthly interval, the beginning, same, or end of the month.
These are the 3 most common ways to use the INTNX function in SAS.
OPTION 1: Add a day/week/month/quarter/year to a date
data work.want; set work.have; add_a_day = intnx("day", start_date, 1, "s"); add_a_week = intnx("week", start_date, 1, "s"); add_a_month = intnx("month", start_date, 1, "s"); add_a_quarter = intnx("qtr", start_date, 1, "s"); add_a_year = intnx("year", start_date, 1, "s"); run;
OPTION 2: Find the first day of the current week/month/quarter/year
data work.want; set work.have; first_day_of_week = intnx("week", start_date, 0, "b"); first_day_of_month = intnx("month", start_date, 0, "b"); first_day_of_quarter = intnx("qtr", start_date, 0, "b"); first_day_of_year = intnx("year", start_date, 0, "b"); run;
OPTION 3: Find the last day of the current week/month/quarter/year
data work.want; set work.have; last_day_of_week = intnx("week", start_date, 0, "e"); last_day_of_month = intnx("month", start_date, 0, "e"); last_day_of_quarter = intnx("qtr", start_date, 0, "e"); last_day_of_year = intnx("year", start_date, 0, "e"); run;
The following examples show how to use the INTNX with a SAS data set.
data work.have; format start_date date9.; input start_date :date9.; datalines; "3JAN2022"d "15MAR2022"d "30APR2022"d "22SEP2022"d "10OCT2022"d ; run; proc print data=work.have; run;
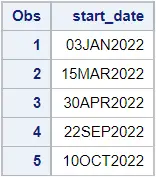
EXAMPLE 1: Add a day/week/month/quarter/year to a date
The following example shows how to add a day/week/month/etc. to a SAS date with the INTNX function.
data work.want; set work.have; add_a_day = intnx("day", start_date, 1, "s"); add_a_week = intnx("week", start_date, 1, "s"); add_a_month = intnx("month", start_date, 1, "s"); add_a_quarter = intnx("qtr", start_date, 1, "s"); add_a_year = intnx("year", start_date, 1, "s"); format add_: date9.; run; proc print data=work.want; run;
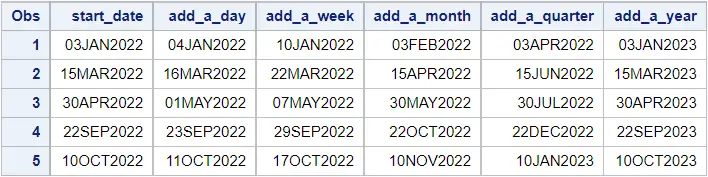
Notice that we’ve added one interval (i.e., a day, week, month, quarter, and year) to the variable start_date.
Instead of adding just one interval, you can use the increment argument also to add multiple intervals to a date. For example, 30 days or 2 months.
Moreover, using a negative value for the increment argument, the SAS INTNX function subtracts one or more intervals (days, weeks, months, etc.) from a date.
For example:
data work.want; set work.have; add_30_days = intnx("day", start_date, 30, "s"); subtract_1_week = intnx("week", start_date, -1, "s"); add_2_months = intnx("month", start_date, 2, "s"); subtract_3_quarters = intnx("qtr", start_date, -3, "s"); add_5_years = intnx("year", start_date, 5, "s"); format add_: subtract_: date9.; run; proc print data=work.want; run;
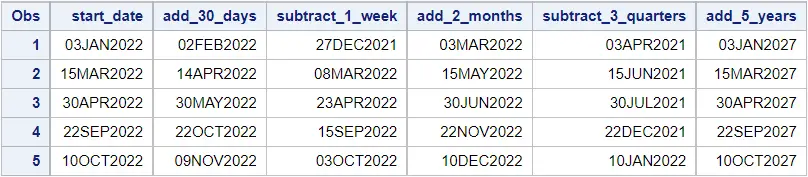
EXAMPLE 2: Find the first day of the current week/month/quarter/year
The following example shows how to find the first day of the current week/month/quarter/year with the SAS INTX function.
You find the first day of a SAS date interval by using “b” (or “beginning”) as the value for the alignment argument.
For example:
data work.want; set work.have; first_day_of_week = intnx("week", start_date, 0, "b"); first_day_of_month = intnx("month", start_date, 0, "b"); first_day_of_quarter = intnx("qtr", start_date, 0, "b"); first_day_of_year = intnx("year", start_date, 0, "b"); format first_: date9.; run; proc print data=work.want; run;
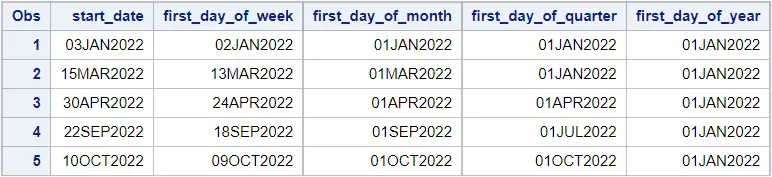
Notice that, by default, in the SAS programming language weeks start on Sunday. For example, January 2nd, 2022, and March 13th, 2022 are both Sundays.
However, you can change this behavior using a different argument for the interval. To let weeks in SAS start on Monday, you can use the “week.2” interval. For weeks starting on Tuesday, you can use “week.3”, etc.
You can also combine the increment argument and the alignment argument to, for example, find the first day of the last month or the first day of the next but one quarter.
For example:
data work.want; set work.have; first_day_next_week = intnx("week", start_date, 1, "b"); first_day_last_month = intnx("month", start_date, -1, "b"); first_day_2_quarter = intnx("qtr", start_date, 2, "b"); first_day_previous_year = intnx("year", start_date, -1, "b"); format first_: date9.; run; proc print data=work.want; run;
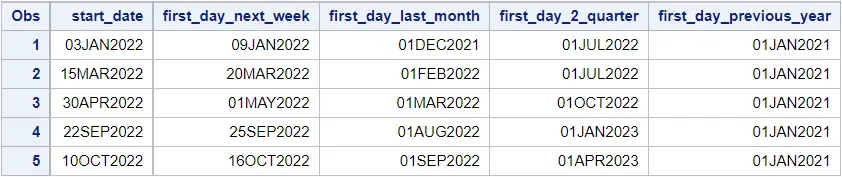
EXAMPLE 3: Find the last day of the current week/month/quarter/year
Lastly, this example shows how to apply the INTNX function to find the last day of the current week/month/quarter/year in SAS.
To find the last day of a given interval in SAS, you need the alignment argument and set it to “e” (or “end”).
For example:
data work.want; set work.have; last_day_of_week = intnx("week", start_date, 0, "e"); last_day_of_month = intnx("month", start_date, 0, "e"); last_day_of_quarter = intnx("qtr", start_date, 0, "e"); last_day_of_year = intnx("year", start_date, 0, "e"); format last_: date9.; run; proc print data=work.want; run;
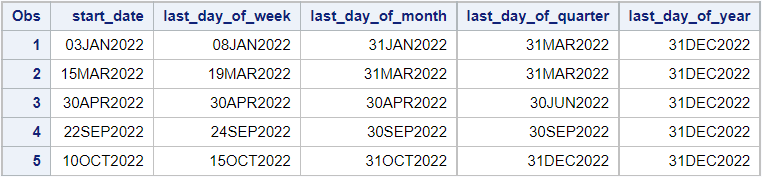
Instead of finding the last date of the current interval, you can also play with the increment argument and find, for example, the last day of the previous month.
For example:
data work.want; set work.have; last_day_next_week = intnx("week", start_date, 1, "e"); last_day_previous_month = intnx("month", start_date, -1, "e"); last_day_2_quarters_ago = intnx("qtr", start_date, -2, "e"); last_day_year_plus_3 = intnx("year", start_date, 3, "e"); format last_: date9.; run; proc print data=work.want; run;
