When you work with numeric data, you sometimes need to round them. In this article, we explain how to use the ROUND-function to round numbers in SAS. Amongst others, we discuss how to round your numbers to the nearest integer and 2 decimals. All examples are supported by images and SAS code.
First, we create a SAS data set with numeric data that we will use in the following sections.
data work.my_data; input my_number; datalines; 2.2 3.99 1.2345 7.876 13.8739 ; run;
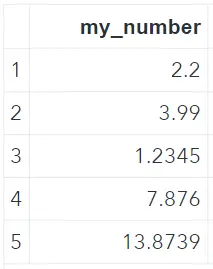
Contents
Rounding to the Nearest Whole Number
The most common way of rounding numbers is rounding to the nearest whole number. That is to say, if the digit at the tenths place is 5 or higher, then round up. If the digit is lower than 5, then round down. For example, SAS round 2.2 to 2 and 3.99 to 4.
The SAS ROUND-function rounds numbers by default to the nearest whole number. You call the ROUND-function and write between the parenthesis the numeric data you want to round. No second argument is required.
/* ROUND DEFAULT */ data work.round_default; set work.my_data; round_default = round(my_number); run;
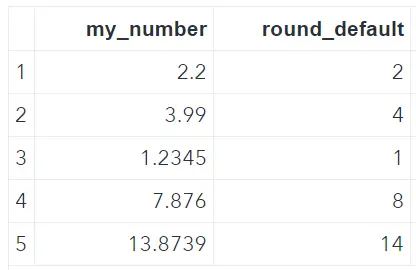
Rounding on Decimals
At first, rounding in SAS to decimal places can be confusing. Luckily, it isn’t that difficult once you understand the trick.
In contrary to rounding to the nearest integer, you need the second argument of the ROUND-function to round to decimal places. In general, the SAS ROUND-function rounds the first argument to the nearest multiple of the second argument. So, if the second argument is 0.1, then SAS rounds to 1 decimal place. If the second argument is 0.01, then SAS round to 2 decimal places, etc.
Below we show how to round to 1st, 2nd, and 3rd decimal places in SAS.
/* ROUNDING WITH DECIMALS */ data work.round_decimals; set work.my_data; round_1_decimal = round(my_number, 0.1); round_2_decimals = round(my_number, 0.01); round_3_decimals = round(my_number, 0.001); run;
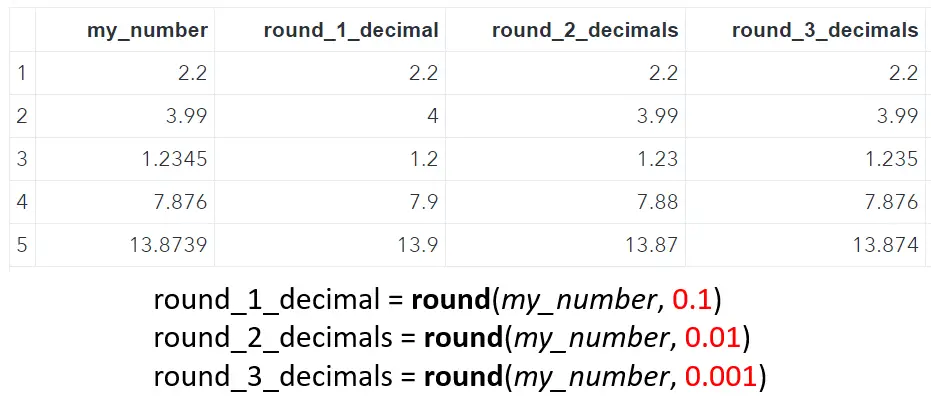
Rounding to the Nearest Multiple
Another common way of rounding is rounding a number to the nearest multiple of 2, 5, 100, etc. So, for example, if you round to the nearest multiple of 10, then 17 will be rounded to 20, and 29 will be rounded to 30.
For rounding to the nearest multiple of a constant in SAS, you need the second argument of the ROUND-function. With the example code below, we demonstrate how to round to the nearest multiple of 2, 3, and 5.
/* ROUNDING TO NEAREST MULTIPLE */ data work.round_nearest_multiple; set work.my_data; round_multiple_2 = round(my_number, 2); round_multiple_3 = round(my_number, 3); round_multiple_5 = round(my_number, 5); run;
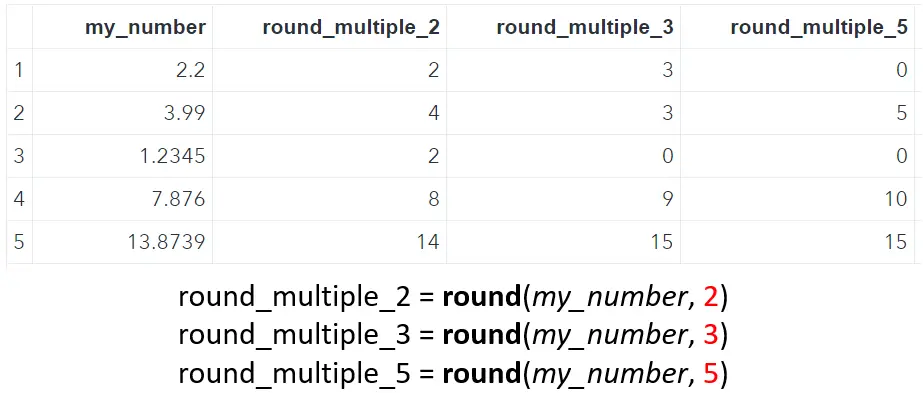
Other Rounding Methods
Other common rounding functions are FLOOR, CEIL, and INT. All three SAS functions take a number as input and don’t require a second argument.
The FLOOR-function rounds its input to the greatest integer less than or equal to the input. So, for example, 3.3 will be rounded to 3, and 6.9 will be rounded to 6.
Contrary to the FLOOR-function, the CEIL-function rounds its input to the smallest integer greater than or equal to the input. That is to say, 3.3 will be rounded to 4, and 6.9 will be rounded to7.
Finally, the INT-function returns the integer part of its input. For example, the INT-function return 3 if the input is 3.3, and 6 if the input is 6.9.
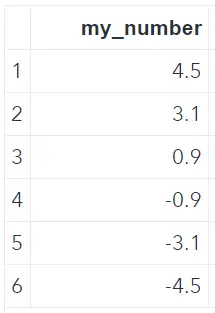
/* FLOOR & CEILING */ data work.my_data2; input my_number; datalines; 4.5 3.1 0.9 -0.9 -3.1 -4.5 ; run; data work.floor_ceiling_int; set work.my_data2; floor = floor(my_number); ceiling = ceil(my_number); int = int(my_number); round= round(my_number); run;
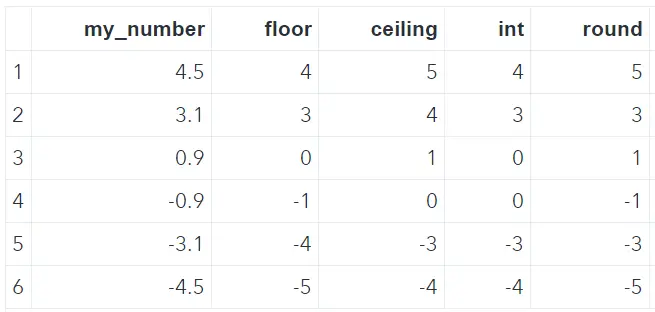
The INT-function returns the same value as the FLOOR-function if the input is positive. If the input is negative the INT-function and the CEIL-function will return the same value.
Find out more about other SAS Functions and SAS How To’s on these pages.
One thought on “How to Round Numbers in SAS”
Comments are closed.