Working with character strings in SAS means dealing with blanks and whitespaces. Very often, these blanks and whitespaces are unwanted, and dealing with them can be a hassle. Luckily, SAS provides many useful, built-in functions to remove blanks. In this article, we discuss the most common functions to remove leading blanks, trailing blanks, and multiple blanks in the middle of a string. We demonstrate how you can apply these functions in your daily work with the use of examples and SAS code.
Contents
Sample Data
Throughout this article, we will use the dataset shown below to demonstrate the differences between the various functions that remove blanks in SAS. This dataset contains a mix of character strings with leading blanks, trailing blanks, and multiple blanks in the middle of the string. Also, the dataset has one string with only blanks.
/* SAMPLE DATA */ data work.cities; input city $char15.; datalines; New York Los Angeles Las Vegas San Diego ; run; data work.sample; set work.cities; string = "*" || city || "*"; run;
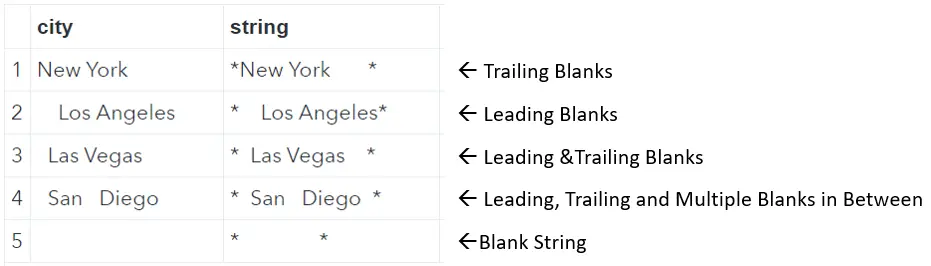
Remove Leading Blanks with the LEFT Function
The first function we discuss to deal with blanks is the LEFT-function. This function only left-aligns character strings and doesn’t remove any blanks. In other words, all blanks are “pushed” to the end of the string. The second string of our example data (“Los Angeles”), makes this very clear.
/* LEFT */ data work.left; set work.cities; string = "*" || city || "*"; left = "*" || left(city) || "*"; drop city; run;
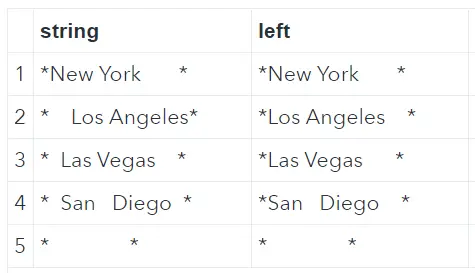
Remove Trailing Blanks with the TRIM Function
The first two functions that actually remove blanks in SAS are the TRIM-function and the TRIMN-function. Both functions remove trailing blanks. However, they differ in how they deal with strings of multiple blanks. If a string consists of only blanks, the TRIM-function returns one blank, while the TRIMN-function returns zero blank characters.
/* TRIM VS. TRIMN */ data work.trim_trimn; set work.cities; string = "*" || city || "*"; trim = "*" || trim(city) || "*"; trimn = "*" || trimn(city) || "*"; drop city; run;
The last string of our example data (the blank string), shows clearly the difference between the TRIM-function and the TRIMN-function.
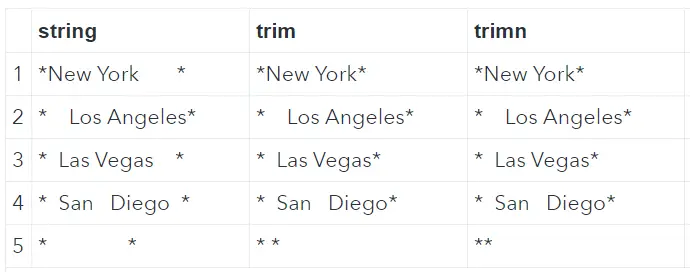
Remove Leading and Trailing Blanks with the STRIP Function
One of the most used functions in SAS to remove blanks is the STRIP-function. Like the TRIM- and TRIMN-functions, the STRIP-function removes trailing blanks. However, the STRIP-function also removes the leading blanks from a string. If the string consists only of blanks, then STRIP-function returns a string of zero blanks.
Instead of using the STRIP-function, you can also use a combination of the LEFT-function and the TRIM(N)-function to remove both leading and trailing blanks. With this combination, you first “push” the leading to the end, and then with the TRIM(N)-function you remove these trailing blanks.
/* STRIP VS. TRIM(LEFT) VS. TRIMN(LEFT) */ data work.strip_trimleft_trimnleft; set work.cities; string = "*" || city || "*"; strip = "*" || strip(city) || "*"; trim_left = "*" || trim(left(city)) || "*"; trimn_left = "*" || trimn(left(city)) || "*"; drop city; run;
The table below shows that the STRIP-function and the combination of the LEFT-function and the TRIMN-function give the same result.
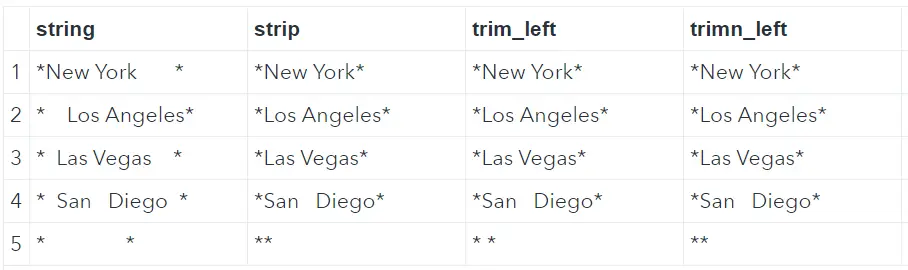
Remove All Blanks with the COMPRESS Function
Finally, if you want to remove all blanks from a string, that is to say, leading blanks, trailing blanks, and blanks within the string, you can use the COMPRESS-function.
/* COMPRESS VS. COMPBL */ data work.compress_compbl; set work.cities; string = "*" || city || "*"; compress = "*" || compress(city) || "*"; compbl = "*" || compbl(city) || "*"; drop city; run;
Another useful function to deal with blanks is COMPBL-function. The COMPBL-function only compresses multiple blanks into one single blank. So, this function is useful to remove unwanted “extra” whitespace within a string.
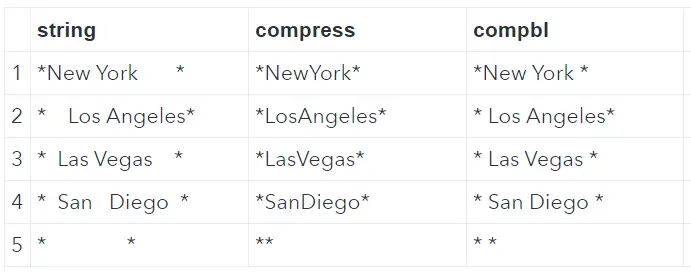
Conclusion
To conclude this article, we show a summary of the most common functions to remove blanks in SAS.
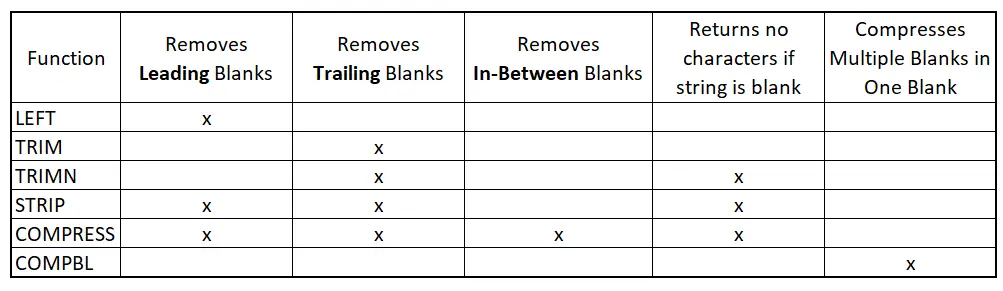
If you struggle with blanks after concatenating string, this article might be useful. In this article, we discuss you to use the CAT-, CATT,- CATS-, and CATX-function. All these functions deal differently with whitespaces.
4 thoughts on “How to Remove Blanks In SAS With Strip, Compress & Trim”
Comments are closed.