Finding the square root is a common operation. However, depending on your programming language the syntax is different. That is why, in this article, we discuss how to calculate the square root in SAS.
In SAS, you calculate the square root of a number with the SQRT function. This function has one argument, namely a number, and returns its square root. Alternatively, you can find the square root by raising a number to 1/2. In SAS, you raise a number to a given power with the double-asterisk (**).
In this article, we show some simple examples of how to use the SQRT function. We calculate the square root in a SAS Data Step and PROC SQL. Also, we demonstrate how to work with the SQRT function in macro variables and macro functions.
Contents
How to Calculate the Square Root in SAS
As mentioned before, you compute the square root of a number by either using the SQRT function or raising it to a half. This is the correct syntax:
The SQRT function
SQRT(<number>)
The power calculation
<number> ** (1/2)
Below we show how to apply these methods in a SAS Data Step and PROC SQL. We will calculate the square root of 1, 2, 4, 9, and 16.
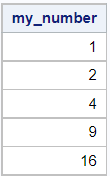
Calculate the Square Root in a SAS Data Step
In the SAS code below, we create new variables that contain the square root of the values in the my_number column. Although we use different methods, the results are the same (as they should be).
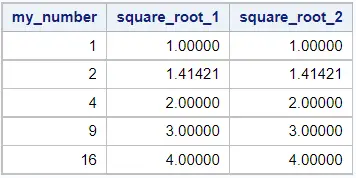
data work.square_root; set work.my_data; square_root_1 = sqrt(my_number); square_root_2 = my_number ** (1/2); run;
Calculate the Square Root in PROC SQL
You can also calculate the square root with PROC SQL. The syntax of the calculation is similar to the SAS Data Step.
proc sql; create table work.square_root as select my_number, sqrt(my_number) as square_root_1, my_number ** 0.5 as square_root_2 from work.my_data; quit;
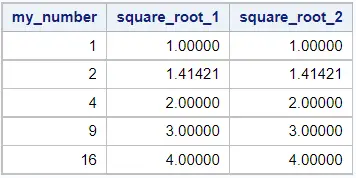
Note that, although a power function exists in the SQL language, you can’t use it in PROC SQL of SAS.
How to Calculate the Cube Root in SAS
Related to the question of how to calculate the square root of a number in SAS is how to calculate the cube root.
Unlike the square root, there is no special function in SAS to calculate the cube root of a number. Therefore, to find the cube root, you need to raise your number to 1/3 with the double-asterisk (**).
Calculate the cube root
<number> ** (1/3)
Below we show some examples with SAS code.
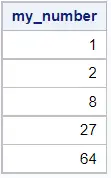
data work.cube_root; set work.my_data_cube; cube_root = my_number ** (1/3); run;
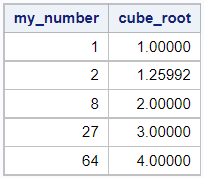
How to Calculate the Square Root in a Macro Variable
Above we’ve answered the question of how to find the square root (and cube root) in a SAS Data Step and the PROC SQL procedure. Another frequently asked question is how to calculate the square root in a SAS Macro Variable or a Macro Function.
You can calculate the square root in a SAS Macro Variable of Function with a combination of the %SYSFUNC function and the SQRT function. Alternatively, you use the %SYSEVAL function and the double-asterisk (**).
In general, SAS functions and mathematical operations don’t work if you are working with macro variables of macro functions. Therefore, if you want to use a function (e.g., the SQRT function), you need to include this function within the %SYSFUNC() code. With this special function, SAS interprets the SQRT as a function instead of normal text.
In the examples below, we demonstrate how to use the %SYSFUNC and SQRT functions to create a macro variable that holds the square root of 3.
%let square_root_9 = %sysfunc(sqrt(9)); %put &=square_root_9;

You can also use a macro variable as an argument for the SQRT function. For example, below we create first a macro variable that holds the value of 16. Then, we use the %SYSFUNC and SQRT functions to calculate its square root.
%let my_number = 16; %let square_root_my_number = %sysfunc(sqrt(&my_number.)); %put &=my_number; %put &=square_root_my_number;
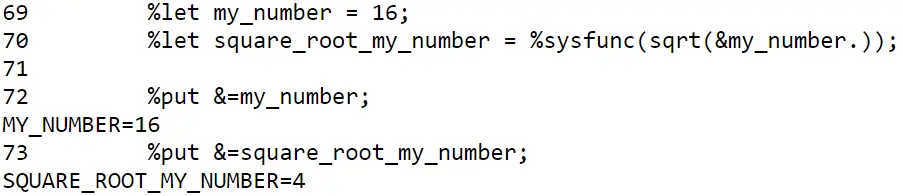
Likewise, you can use the %SYSEVAL function and the double-asterisk (**) to find the square root of a number. With the %SYSEVAL function, SAS interprets the double-asterisk as a mathematical operation instead of plain text. Below we show two examples
%let square_root_9 = %sysevalf(9 ** (1/2)); %put &=square_root_9;

%let my_number = 16; %let square_root_my_number = %sysevalf(&my_number. ** (1/2)); %put &=my_number; %put &=square_root_my_number;
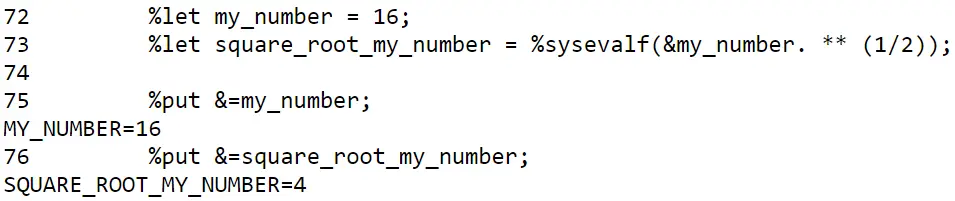