Working with dates is one of the tasks that cause the most frustration while learning SAS. For example, calculating the difference between two dates, adding a month to a date, or converting a number to a SAS date.
However, if you happen to have a numeric variable and you want to convert it into a SAS date, it’s actually pretty easy. It just requires 3 simple steps.
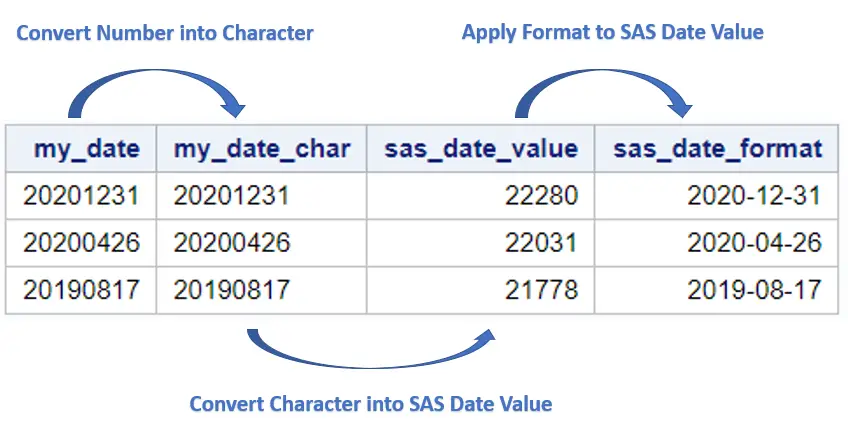
Before we show how to create a SAS date from a number, we first discuss briefly what SAS dates are and how to interpret them. This way, you will find it easier to understand these 3 steps (and will never forget them, hopefully).
Note, if you have a text string that looks like a date, then we recommend reading this article.
Contents
What are SAS Dates?
A date value in SAS represents the number of days between January 1st, 1960, and a specified date. Dates before January 1st, 1960 are negative numbers, while dates after January 1st, 1960 are positive numbers.
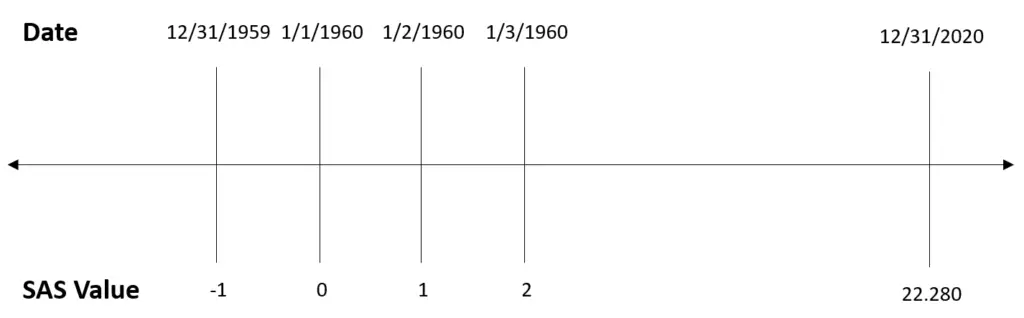
It is important to understand that a numeric value in SAS that looks like a date isn’t equal to the SAS value that represents this date. For example, the value 12312020 doesn’t represent December 31, 2020. See the image above.
If you consider that you can write December 31, 2020, in many ways (12312020, 31122020, 20201231, etc.), it becomes clear that numeric values that look like dates can’t be the SAS values that represent these dates.
So, if you have a number and want to use it as a date, you need to convert it into a SAS date first. Below we show how to create SAS dates from the values in the my_date column with just 3 simple steps.
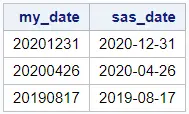
How to Convert a Number to a SAS Date?
1. Convert the Number to a Character String
The first step to create a SAS date from a number is to convert the number into a character string. You can convert a number into a character string with the PUT function.
PUT(numeric-value, format)
The PUT function takes as an argument a numeric value and returns a character string. The second argument, i.e., the SAS format, specifies how SAS must interpret the numeric value. In this case, we use the 8. format.
/* Step 0. Sample Data */ data work.my_ds; input my_date; datalines; 20201231 20200426 20190817 ; run; /* Step 1. Convert Numeric to Character */ data work.my_ds; set work.my_ds; my_date_char = put(my_date, 8.); run;
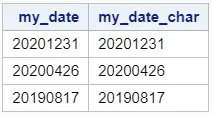
You can use the PROC CONTENTS procedure to check that the new column my_date_char is a character string.
proc contents data=work.my_ds varnum; run;
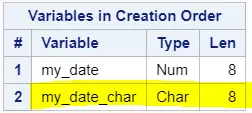
2. Convert the Character String to a SAS Date Value
The second step is to convert the character string into a SAS date value. In other words, convert the character string into the SAS value that represents the date.
You can convert a character string into a SAS date with the INPUT function. The INPUT function has two arguments, namely the character string and an informat, and return the value that represents the date in SAS.
INPUT(character-value, informat)
The informat lets SAS know you to interpret the character string. Because the character string in our example is 8 character longs and looks like “year-month-day”, we use the yymmdd8. informat.
data work.my_ds; set work.my_ds; my_date_char = put(my_date, 8.); sas_date_value = input(my_date_char, yymmdd8.); run; proc print data=work.my_ds noobs; run;
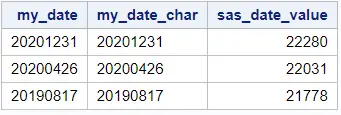
Note that, because not all dates are written as “year-month-day”, you might need to change the informat. See below a list of the most common informats.
If you don’t know which format to use in the INPUT function, you can always try the ANYDTDTE8. format. This format makes an educated guess to convert the character string into a SAS date value.
3. Apply a Format to the SAS Date Value
The last step is to apply a SAS Date Format to the SAS Date Value.
As you can see in the image above, the sas_date_value column contains values that represent SAS dates. However, humans can interpret these values as dates. So, we need to apply a format to make them readable.
You use the FORMAT statement to apply a format to a variable.
There exist many formats in SAS to make a date value readable. We will use the YYMMDD10. format.
data work.my_ds; set work.my_ds; my_date_char = put(my_date, 8.); sas_date_value = input(my_date_char, yymmdd8.); sas_date_format = sas_date_value; format sas_date_format yymmdd10.; run; proc print data=work.my_ds noobs; run;
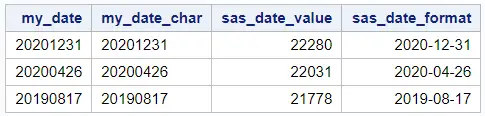
Above we used 3 steps to make clear how to convert a number into a SAS date. However, you could use only one step instead.
data work.my_ds; input my_date; sas_date = input(put(my_date, 8.), yymmdd8.); format sas_date yymmdd10.; datalines; 20201231 20200426 20190817 ; run; proc print data=work.my_ds noobs; run;
In the next section, we present more SAS Date formats.
What are SAS Formats?
As mentioned before, a SAS date is a numeric value that represents the number of days between January 1st, 1960 and a specific date. To make this numeric value interpretable for humans, you need to apply a SAS Date Format.
There exist many SAS Date formats. Below we show a table with the most common formats.
You can use these formats with the INPUT function and the FORMAT statement.
SAS Format | Formatted Value |
---|---|
yymmdd10. | 2020-12-31 |
yymmddb10. | 2020 12 31 |
yymmddp10. | 2020.12.31 |
yymmdds10. | 2020/12/31 |
yymmddc10. | 2020:12:31 |
yyddmm10. | 2020-31-12 |
yyddmmb10. | 2020 31 12 |
yyddmmp10. | 2020.31.12 |
yyddmms10. | 2020/31/12 |
yyddmmc10. | 2020:31:12 |
mmddyy10. | 12-31-2020 |
mmddyyb10. | 12 31 2020 |
mmddyyp10. | 12.31.2020 |
mmddyys10. | 12/31/2020 |
mmddyyc10. | 12:31:2020 |
ddmmyy10. | 31-12-2020 |
ddmmyyb10. | 31 12 2020 |
ddmmyyp10. | 31.12.2020 |
ddmmyys10. | 31/12/2020 |
ddmmyyc10. | 31:12:2020 |
yymmdd8. | 20201231 |
yyddmm8. | 20203112 |
mmddyy8. | 12312020 |
ddmmyy8. | 31122020 |
In general, to convert a numeric value into a SAS date, you need the YYMMDD8., YYDDMM8., MMDDYY8., or DDMMYY8. format.
5 thoughts on “How to Easily Convert a Number to a Date in SAS”
Comments are closed.