One of the good programming practices regarding variable names is to avoid the use of blanks and special characters. In fact, SAS doesn’t allow you to use them in the first place and limits the number of characters to 32. However, if you create a report, it might be necessary to have more flexibility with respect to the length and the (special) characters you can use for your variables.
For this reason, SAS introduced variable labels.
In this article, we discuss what variable labels are, how to create, modify, and delete them, and how to display them.
Contents
What are Labels in SAS
A SAS variable label is a text string of up to 256 (special) characters that you can use in reports and SAS procedures. A variable label gives you flexibility in terms of length and typographical characters that you can use.
In other words, while a SAS variable name has a maximum length of 32 characters and cannot contain special characters, a variable label can have up to 256 letters, numbers, spaces, percentage-signs, etc.
How to Display all Variable Labels in SAS
Before we explain how to create, modify, and remove a variable label, it is useful to understand how to display the current labels of the variables in your dataset. But, how do you display all variable labels?
You use PROC CONTENTS to display the permanent labels that are assigned the variables in your specific dataset.
If you run the example code below, SAS displays the labels of all variable in the HEART dataset from the SASHELP library.
proc contents data=sashelp.heart; run;
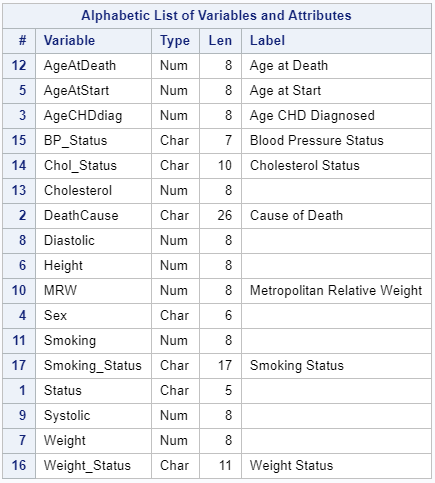
As you can see, PROC CONTENTS provides you with a list of variable names and their labels (if any).
You can run this piece of SAS code before and after you create, modify, or remove a label to check if you obtain the desired result.
How to Create a Variable Label in SAS
In SAS, you can create a variable label with the LABEL statement. You can use this statement to assign one or more labels using 3 methods, namely a SAS DATA Step, the PROC SQL procedure, and the PROC DATASETS procedure.
The exact syntax of the LABEL statement depends on the method of choice.
Next, we explain the 3 methods in more detail, but first we create a simple dataset with test scores to illustrate that we will use in the examples.
data work.my_ds; input Student $ Course $ Mark; datalines; Max Physics 90 Angie French 88 Josh Biology 85 ; run;
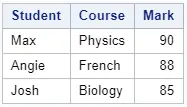
If you run the CONTENTS procedure, SAS shows that our sample dataset doesn’t have permanent variable labels yet.
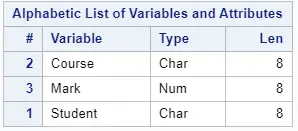
Create a Variable Label in a SAS DATA Step
The first method to assign a label to a variable in SAS is with a DATA Step.
To create a variable label in a SAS DATA Step you need the LABEL keyword, followed by the variable name to which you want to assign a label, an equal sign, and the desired label between single quotes.
With the code below we assign the label Student Name to the Student column.
data work.create_label; set work.my_ds; label Student = 'Student Name'; run;
We recommend to place the LABEL statement after the SET statement. Otherwise, if you place the LABEL statement before the SET statement, the structure of the output table changes.
Do you know? How to Change the Structure of a Table Properly
Create a Variable Label with PROC SQL
The second method to create a variable label is with the PROC SQL procedure.
If you use PROC SQL to create a label, you place the LABEL statement directly after the variable name. The LABEL statement is followed by an equal sign and the desired label between quotes.
With the code below we assign the label Student Name to the Student column.
proc sql; create table work.create_label as select student label='Student Name', course, mark from work.my_ds; quit;
With this method, it’s necessary to specify all column names, even though you want to create just one label. Consequently, if your table has many columns, this can be a tedious task. Hence, we only recommend this method if your table has few columns.
Create a Variable Label with PROC DATASETS
The third method to create a variable label in SAS is with PROC DATASETS.
You use the PROC DATASETS procedure in combination with the LABEL statement to assign a label to a variable in SAS. The syntax of the LABEL statement is straightforward. The LABEL keyword is followed by the name of the variable, an equal sign, and the label between quotes.
With the code below we assign the label Student Name to the Student column.
proc datasets lib=work; modify my_ds; label student = 'Student Name'; run;
With this method you can only modify the specified dataset. Hence, it isn’t possible to create a new dataset.
By default, SAS creates a report when you run the PROC DATASETS procedure. If you want to suppress this report, you can add the NOLIST option after the LIB option.
How to Label Multiple Variables
In the examples above, we have provided three methods to assign a label to one variable. But, how do you create labels for multiple variables?
Instead of repeating the code above several times, you can change the code slightly to add labels to multiple variables at once. The syntax is intuitive and doesn’t need much explanation.
The examples below show how to assign labels to variables for each of the three methods. We create labels for the variables Student and Course.
/* DATA Step */ data work.create_label; set work.my_ds; label Student = 'Student Name' Course = 'Course Name'; run; /* PROC SQL */ proc sql; create table work.create_label as select student label='Student Name', course label='Course Name', mark from work.my_ds; quit; /* PROC DATASETS */ proc datasets lib=work; modify my_ds; label Student = 'Student Name' Course = 'Course Name'; run;
How to Change a Variable Label in SAS
So far, we’ve demonstrated how to add a label to a variable. But, how do you change a variable label in SAS?
Changing a variable label in SAS is exactly the same as assigning a label. SAS doesn’t require you to specify the old label before you can define the new label. So, you can directly use the LABEL statement to modify the current label.
As an example, we create a dataset of 3 columns of which 2 have a column label (Student and Course). We use PROC CONTENTS to show the labels.
data work.my_ds; input Student $ Course $ Mark; label Student = 'Student Name' Course = 'Course Name'; datalines; Max Physics 90 Angie French 88 Josh Biology 85 ; run; proc contents data=work.my_ds; run;
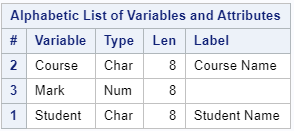
Now, we use 3 methods (a SAS DATA Step, PROC SQL, and PROC DATASETS) to modify the existing column labels.
/* DATA Step */ data work.change_label; set work.my_ds; label Student = 'First Name' Course = 'Class'; run; /* PROC SQL */ proc sql; create table work.change_label as select student label = ' First Name', course label = 'Class', mark from work.my_ds; quit; /* PROC DATASETS */ proc datasets lib=work; modify my_ds; label Student = 'First Name' Course = 'Class'; run; proc contents data=work.change_label; run;
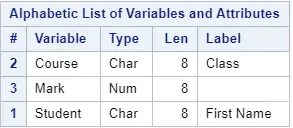
As you can see, we have successfully changed the labels of the Student and Course column.
Do you know? How to Modify Variable Names
How to Remove a Variable Label in SAS
Although you normally want to create or modify a variable label, you can also remove a label. You delete a label with the LABEL statement in a DATA Step, in PROC SQL, or in PROC DATASETS.
Below we show 3 different ways to remove the permanent label of the Species variable from the IRIS dataset in the SASHELP library.
Remove a Variable Label in a SAS DATA Step
The first method to remove a variable label is with a SAS DATA Step and the LABEL statement. After the LABEL keyword, you specify the variable name from which you want to remove the label and an equal sign.
The DATA Step below removes the label of the Species variable.
data work.remove_label; set sashelp.iris; label species =; run;
Note: You could please the LABEL statement before the SET statement in order to delete the label. However, this will change the order of columns in the output dataset.
Do you know? How to Reorder Columns in SAS
Remove a Variable Label with PROC SQL
The second method to delete a variable label in SAS is with PROC SQL.
To remove the label with PROC SQL you specify the variable name, the LABEL keyword, an equal sign, and two quotes without a blank.
The SAS code below removes the label from the SPECIES variable.
proc sql; create table work.remove_label as select Species label='', SepalLength, SepalWidth, PetalLength, PetalWidth from sashelp.iris; quit;
Compared to the first method, this method has the drawback that you always need to write out all variables names even if you want to remove just one label.
Remove a Variable Label with PROC DATASETS
The third method to remove variable labels is with PROC DATASETS.
The first step to remove the label with PROC DATASETS is to provide the library name where your dataset is located. Then, you use the MODIFY statement to specify the dataset in which you want to remove a label. Finally, you use the LABEL keyword followed by the variable name and an equal sign.
The code below removes the label of the Species variable with PROC DATASETS.
data work.iris; set sashelp.iris; run; proc datasets lib=work; modify iris; label Species=; run;
Note: With this method you remove the label from the original dataset because of the LIB option. It is not possible to create a new dataset without the variable label.
Do you know? 10 Things You Should Know About SAS Libraries
Remove Multiple Variable Labels
In the examples above we have removed one variable label at a time. However, how do you remove multiple variables labels at once?
You can use three methods (a SAS DATA Step, PROC SQL, and PROC DATASETS) in combination with the LABEL statement to remove multiple variables at the same time. With a SAS DATA Step and PROC DATASETS you need the LABEL keyword, followed by the variable names from which you want to remove the label, and an equal sign. If you use PROC SQL, you need a separate LABEL statement for each variable.
Below we provide an example of each method. We remove the labels from the variables Species and SepalWidth.
/* DATA Step */ data work.remove_label; set sashelp.iris; label species = sepalwidth=; run; /* PROC SQL */ proc sql; create table work.remove_label as select Species label='', SepalLength, SepalWidth label='', PetalLength, PetalWidth from sashelp.iris; quit; /* PROC DATASETS */ data work.iris; set sashelp.iris; run; proc datasets lib=work; modify iris; label Species= SepalWidth=; run;
Remove All Variable Labels
Lastly, it is also possible to remove all variable labels at once.
The best method to remove all labels is PROC DATASETS, in combination with the ATTRIB option. A SAS DATA Step and PROC SQL are less suited for this purpose because you have to explicitly mention all variables. Instead, PROC DATASETS is a more robust method to remove all variable labels.
Contrary to the methods mentioned above to remove one (or more) labels, you don’t need the LABEL statement to remove efficiently all labels. Instead, you need the ATTRIB option with the LABEL option.
To remove all labels from your variables, you write the ATTRIB keyword, followed by the special keyword _ALL_ (to refer to all variables), and the LABEL option. The LABEL option you define as two single quotes without whitespace.
With the code below you can delete all permanent variable labels from the Iris dataset.
data work.iris; set sashelp.iris; run; proc datasets lib=work; modify iris; attrib _all_ label=''; run;
What is the Difference between the LABEL Statement and LABEL Option
Thus far, we have created, modified, and removed SAS variable labels with the LABEL statement. However, there exists also a LABEL option. So, what is the difference between the LABEL statement and the LABEL option?
The difference between the LABEL statement and the LABEL option is its usage. While the LABEL statement can be used to create, modify, and remove variable labels, the LABEL option can be applied to show the labels. Also, the LABEL option can be used as a data set option to create a label for a data set.
In the remainder of this article, we will demonstrate how to use the LABEL option to show and export variable labels instead of variable names.
How to Show Variable Labels in SAS
As mentioned before, labels are primarily used in presenting results. However, so far, we have only demonstrated how to create, modify, and remove labels. So, how do you show variable labels in a SAS report or an export procedure?
You use the LABEL option to show the variable labels in your reports. This option overrides the default behavior of showing the variable names. You can use this option in many procedures, such as PROC PRINT, PROC MEANS, and PROC EXPORT.
How to Show Labels in PROC PRINT
Variable labels are commonly used for presenting results. If you want to present a SAS table, then you can use the PROC PRINT procedure. By default, this procedure presents the column names. So, how do you show the column labels with PROC PRINT?
You show the column labels instead of the column names in a PRINT procedure by adding the LABEL option.
Below we show the difference between the default behavior of PROC PRINT and the result of adding the LABEL option. We print the first 6 rows of the columns Make, Model, EngineSize, and Weight from the CARS dataset in the SASHELP library.
The code and image below show the default behaviour of PROC PRINT (i.e., no variable labels).
proc print data=sashelp.cars (keep = Make Model EngineSize Weight obs=6); run;
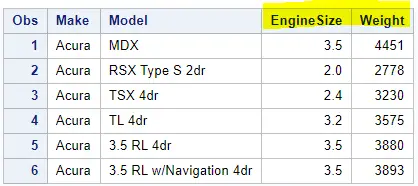
In the code below we add the LABEL option to show the variable labels instead of the variable names in the output of PROC PRINT.
proc print data=sashelp.cars (keep = Make Model EngineSize Weight obs=6) label; run;
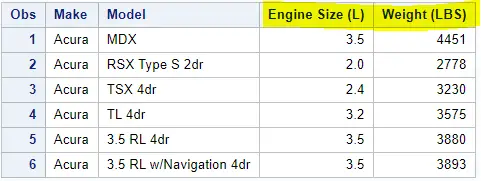
By default, the PROC PRINT procedure prints rows numbers (in the Obs column). If you want a report without row numbers, you can use the NOOBS option.
Do you know? How to Select the First N Rows of a Table and How to Use the KEEP Option to Select Columns?
It is also possible to add a temporary label to a variable with PROC PRINT. Temporary variable labels are labels that only exist during the execution of a specific procedure. When the execution of the procedure has finished, these temporary labels disappear.
For example, the Make and Model column in the CARS dataset don’t have permanent labels. Here we use a LABEL statement to assign them a temporary label.
Notice that in the code below we use the LABEL option as well as the LABEL statement.
proc print data=sashelp.cars (keep = Make Model EngineSize Weight obs=6) label; label Make = 'Vehicle Make' Model = 'Vehicle Model'; run;
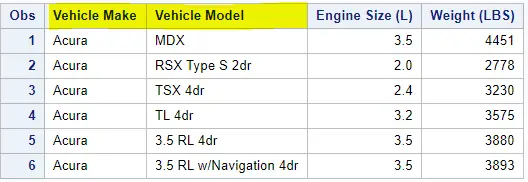
Note: You can also use temporary labels to modify temporarily permanent labels.
How to Show Labels in PROC FREQ
Another way of presenting results is with PROC FREQ. With this procedure, you can create frequency tables of a specific variable. If this variable doesn’t have a permanent label or you want to modify it temporarily, you can add a temporary label. But, how do you show a temporary label in PROC FREQ?
You can add a temporary label to the output of the PROC FREQ procedure with the LABEL statement.
In the example below we show how to add a temporary label to the variable Make from the CARS dataset.
proc freq data=sashelp.cars; tables Make; run;
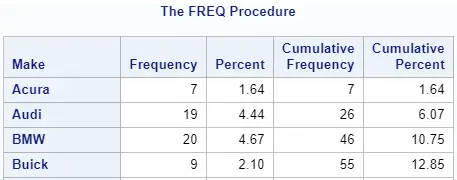
Now we use the LABEL statement to add a temporary label to the Make variable
proc freq data=sashelp.cars; tables Make; label Make = 'Cars Make'; run;
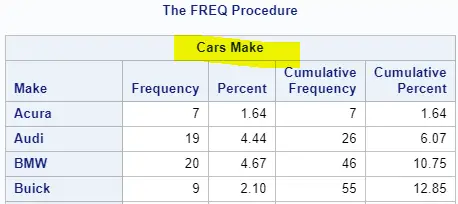
In PROC FREQ there doesn’t exist an option to prevent SAS from printing the variable label. So, if you want a frequency table without a label, you need to add the LABEL statement to your code and define the label as two single quotes (without blanks).
Do you know? How to Get the Most Out of PROC FREQ
How to Show Labels in PROC EXPORT
A third procedure that is frequently used in SAS is PROC EXPORT. You can use this procedure to export a SAS dataset in different formats, e.g., Excel, CSV, or TXT. However, by default, this procedure exports the column names. So, how do you export column labels instead with PROC EXPORT?
You can use the LABEL option in the PROC EXPORT procedure to show the column labels in the output file. If the column doesn’t have a permanent label, PROC EXPORT will show the column name instead.
Here we show how to use the LABEL option in PROC EXPORT.
/* XLSX */ proc export data=sashelp.cars outfile="/folders/myfolders/export/cars.xlsx" dbms=xlsx label; run; /* CSV */ proc export data=sashelp.cars outfile="/folders/myfolders/export/cars.csv" dbms=csv label; run; /* TXT */ proc export data=sashelp.cars outfile="/folders/myfolders/export/cars.txt" dbms=tab label; run;
Are you an expert in PROC EXPORT? Read these articles about exporting your data as XLSX, CSV, and TXT.
How to Not Show Labels in PROC MEANS
Another frequently used procedure in SAS to show results is PROC MEANS. This procedure is one of the few procedures that does show variable labels by default. However, sometimes you don’t want to show them. So, how do you remove variable labels in PROC MEANS?
You remove variable labels from the PROC MEANS procedure by using the NOLABELS option. In the examples below we show how to use this option and the results.
With this code SAS does show the variable labels in the PROC MEANS output.
proc means data=sashelp.cars; var EngineSize Weight; run;

Here we add the NOLABEL option to prevent PROC MEANS from printing the variable labels.
proc means data=sashelp.cars nolabels; var EngineSize Weight; run;
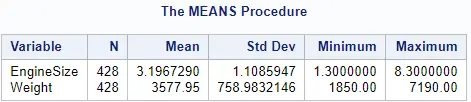
What is the Difference between Permanent and Temporary Labels in SAS
There exist 2 types of variable labels in SAS, namely permanent labels and temporary labels. Both have their purpose, but what is the difference between a permanent and temporary label?
A permanent variable label is a label that is permanently assigned to a variable. You can check if a variable has a permanent variable and its values with PROC CONTENTS. In contrast, a temporary label only exists during the execution of a specific procedure like PROC PRINT or PROC MEANS.
The result of the SAS code below learns us that the Make and Model column in the CARS dataset from the CARS library don’t have a permanent label. However, the EngineSize and Weight columns do have a permanent label.
proc contents data=sashelp.cars; run;
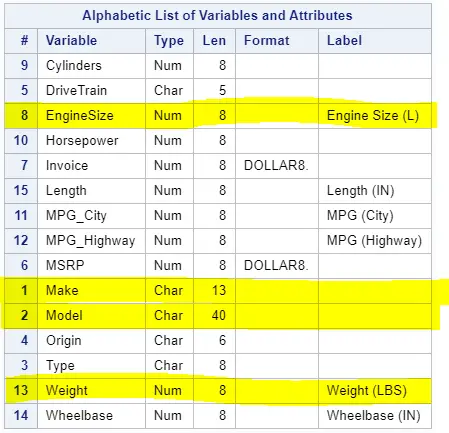
Here we print the first 6 rows of the CARS dataset. We use the LABEL statement to assign the columns Make and Model a temporary label. These labels only exist when the PROC PRINT procedure runs.
proc print data=sashelp.cars (keep=Make Model EngineSize Weight obs=6) label; label Make = 'Cars Make' Model = 'Cars Model'; run;
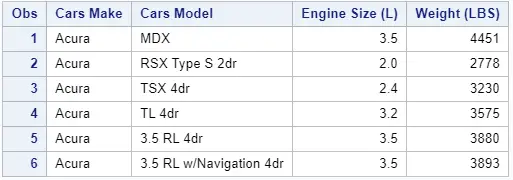
4 thoughts on “How to Label Variables in SAS”
Comments are closed.